Dot Net Interview Questions & Answer
Introduction of dot net interview questions:
Dot Net Core 7 is the latest version of the .NET Core framework, a cross-platform, open-source development framework by Microsoft. It empowers developers to build modern, high-performance applications for web, mobile, cloud, gaming, IoT, and more. As companies embrace .NET Core 7 for their projects, the demand for skilled developers is soaring. This article aims to equip you with the knowledge and confidence to tackle Dot Net interview questions with ease.
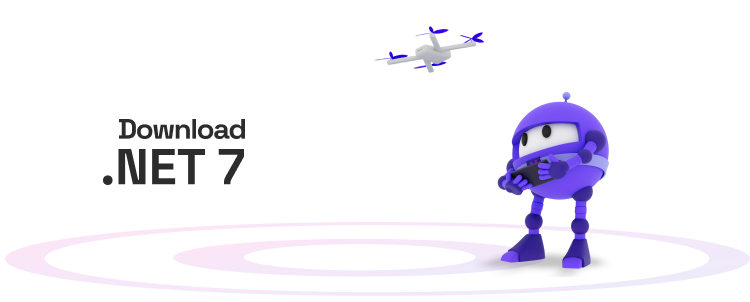
Dot NET Core 7 interview Questions
1. What are the Key Features of Dot Net Core 7?
Example: One of the key features of Dot Net Core 7 is the introduction of nullable reference types. This feature allows developers to annotate variables and parameters as nullable or non-nullable, reducing the risk of null reference exceptions. For example:
public string? GetFullName(string firstName, string? lastName)
{
return lastName != null ? $"{firstName} {lastName}" : firstName;
}
In this example, the lastName
parameter is marked as nullable, and the method safely handles null values.
2. How Does Dot Net Core 7 Differ from Previous Versions?
Example: Dot Net Core 7 introduces enhanced support for Source Generators, a new feature that allows you to generate C# source code at compile-time. For instance:
[MyCustomAttribute]
public class MyClass
{
// Your class implementation here
}
public class MyCustomAttribute : Attribute { }
In this example, the Source Generator processes the MyClass
type and generates additional code based on the presence of the MyCustomAttribute
.
3. What Are the Advantages of Using Dot Net Core 7 for Cross-Platform Development?
Example: With Dot Net Core 7, you can develop cross-platform applications with ease. For example, consider a web application that needs to run on both Windows and Linux servers. Dot Net Core 7 allows you to publish the application as a self-contained deployment, ensuring it runs efficiently on both platforms without any platform-specific modifications.
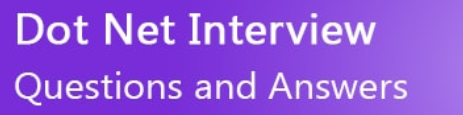
4. How Does the Dot Net Core 7 CLI Facilitate the Development Process?
Example: The Dot Net Core 7 CLI provides a simple and powerful way to manage projects and build applications. For instance, creating a new project can be done with a single command:
bash Copy code
dotnet new console -n MyConsoleApp
This command creates a new console application named MyConsoleApp
.
5. What Are Self-Contained and Framework-Dependent Deployments in Dot Net Core 7?
Example: When deploying a Dot Net Core 7 application, you have two options: self-contained and framework-dependent deployments. For example, when deploying a web application, you can use a self-contained deployment to bundle the .NET Core runtime with your application, making it independent of the system’s installed runtime.
This article “Dot NET Core 7 Interview Questions” should be helpful for *increase the .NET Core knowledge
6. What Is the Role of ASP.NET Core 7 in Dot Net?
Example: ASP.NET Core is a versatile framework in Dot Net Core 7 for building web applications. For example, consider creating a basic API using ASP.NET Core:
csharp Copy code
[Route("api/[controller]")]
[ApiController]
public class SampleController : ControllerBase
{
[HttpGet]
public IActionResult Get()
{
return Ok("Hello, Dot Net Core 7!");
}
}
In this example, the SampleController
responds with a simple message when accessed via HTTP GET.
7. How Does Dependency Injection Work in ASP.NET Core?
Example: Dependency Injection (DI) is a powerful pattern in ASP.NET Core, enhancing code maintainability and testability. For instance, consider a service that calculates the total price of a shopping cart:
This is the most important dot net interview question
csharp Copy code
public class ShoppingCart
{
private readonly IProductService _productService;
public ShoppingCart(IProductService productService)
{
_productService = productService;
}
public decimal CalculateTotalPrice(IEnumerable<string> productIds)
{
decimal totalPrice = 0;
foreach (var productId in productIds)
{
var productPrice = _productService.GetProductPrice(productId);
totalPrice += productPrice;
}
return totalPrice;
}
}
In this example, the ShoppingCart
class uses constructor injection to receive an instance of the IProductService
interface, allowing it to calculate the total price of the products in the shopping cart.
8. What Are Middleware in ASP.NET Core, and How Do They Impact the Request Pipeline?
Example: Middleware components in ASP.NET Core process HTTP requests and responses. For example, let’s create a custom middleware that logs each request’s URL:
This is the most important Dot Net Core 7 Interview questions
csharp Copy code
public class RequestLoggerMiddleware
{
private readonly RequestDelegate _next;
public RequestLoggerMiddleware(RequestDelegate next)
{
_next = next;
}
public async Task Invoke(HttpContext context)
{
var requestUrl = context.Request.Path;
Console.WriteLine($"Request URL: {requestUrl}");
await _next(context);
}
}
In this example, the RequestLoggerMiddleware
logs the request URL before passing it to the next middleware in the pipeline.
9. Exploring Hosting Models in ASP.NET Core: In-Process vs. Out-of-Process
Example: ASP.NET Core supports two hosting models: in-process and out-of-process. For example, when hosting an ASP.NET Core application on IIS, you can choose either the in-process hosting model, where the application runs within the IIS worker process, or the out-of-process model, where the application runs in a separate process.
10. How Does Kestrel Contribute to the ASP.NET Core Web Server Ecosystem?
Example: Kestrel is the web server used by default in ASP.NET Core. For example, when running a web application with Kestrel, you can customize its options, such as configuring the server to listen on specific ports or binding to specific IP addresses.
csharp Copy code
public class Program
{
public static void Main(string[] args)
{
var host = new HostBuilder()
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseKestrel(options =>
{
options.Listen(IPAddress.Loopback, 5000); // Listen on localhost:5000
});
webBuilder.UseStartup<Startup>();
})
.Build();
host.Run();
}
}
In this example, the application is configured to listen on localhost:5000
using Kestrel.
11. How to Secure Your Dot Net Core 7 Applications: Best Practices and Security Mechanisms
Example: Securing Dot Net Core 7 applications is crucial to protect against vulnerabilities. For example, consider implementing authentication and authorization using ASP.NET Core Identity:
csharp Copy code
services.AddIdentity<ApplicationUser, IdentityRole>()
.AddEntityFrameworkStores<ApplicationDbContext>()
.AddDefaultTokenProviders();
In this example, ASP.NET Core Identity is configured to manage user accounts and roles.
12. Understanding Razor Pages in Dot Net Core 7: The Evolution of MVC
Example: Razor Pages is a new feature in Dot Net Core 7 that simplifies the development of web pages. For example, create a Razor Page to display a list of products:
- I am try to collect the best Dot Net Interview Questions
html Copy code
@page
@model ProductModel
<h2>Product List</h2>
<ul>
@foreach (var product in Model.Products)
{
<li>@product.Name</li>
}
</ul>
In this example, the Razor Page displays a list of products retrieved from the ProductModel
class.
13. Leveraging Entity Framework Core in Dot Net Core 7 for Database Access
Example: Entity Framework Core is a powerful ORM in Dot Net Core 7 for database access. For example, create a simple data model and perform CRUD operations:
csharp Copy code
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
// Perform CRUD operations
var product = new Product { Name = "Sample Product", Price = 19.99 };
context.Products.Add(product);
context.SaveChanges();
In this example, a Product
entity is created and persisted to the database using Entity Framework Core.
14. How to Handle and Log Errors Effectively in Dot Net Core 7 Applications
Example: Error handling and logging are vital for a robust application. For example, implement a custom exception filter to handle and log exceptions:
csharp Copy code
public class CustomExceptionFilter : IExceptionFilter
{
public void OnException(ExceptionContext context)
{
var exception = context.Exception;
// Log the exception details
Console.WriteLine($"An exception occurred: {exception.Message}");
}
}
In this example, the CustomExceptionFilter
logs any exceptions that occur during the request processing.
15. Exploring Tag Helpers in ASP.NET Core: Enhancing View Components
Example: Tag Helpers are a powerful feature in ASP.NET Core for creating reusable and clean view components. For example, create a custom tag helper to display formatted dates:
html Copy code
<!-- Usage in Razor view -->
<my-date value="DateTime.Now"></my-date>
<!-- Custom Tag Helper implementation -->
[HtmlTargetElement("my-date")]
public class MyDateTagHelper : TagHelper
{
[HtmlAttributeName("value")]
public DateTime Value { get; set; }
public override void Process(TagHelperContext context, TagHelperOutput output)
{
output.TagName = "span";
output.Content.SetContent(Value.ToShortDateString());
}
}
In this example, the MyDateTagHelper
formats the provided date and renders it as a span element.
16. Understanding the .csproj File Format in Dot Net Core 7 Projects
Example: The .csproj file is a central part of a Dot Net Core 7 project. For example, add a NuGet package reference in the .csproj file:
xml Copy code
<ItemGroup>
<PackageReference Include="Newtonsoft.Json" Version="13.0.1" />
</ItemGroup>
In this example, the Newtonsoft.Json NuGet package is added as a dependency to the project.
17. What’s New in Dot Net Core 7: A Closer Look at the Latest Updates and Improvements
Example: Dot Net Core 7 introduces various new features and improvements. For example, take a closer look at the new Nullable
attributes introduced in C# 8:
csharp Copy code
#nullable enable
public class Person
{
public string FirstName { get; set; }
public string? MiddleName { get; set; }
public string LastName { get; set; }
}
In this example, the MiddleName
property is marked as nullable, indicating that it can have a null value.
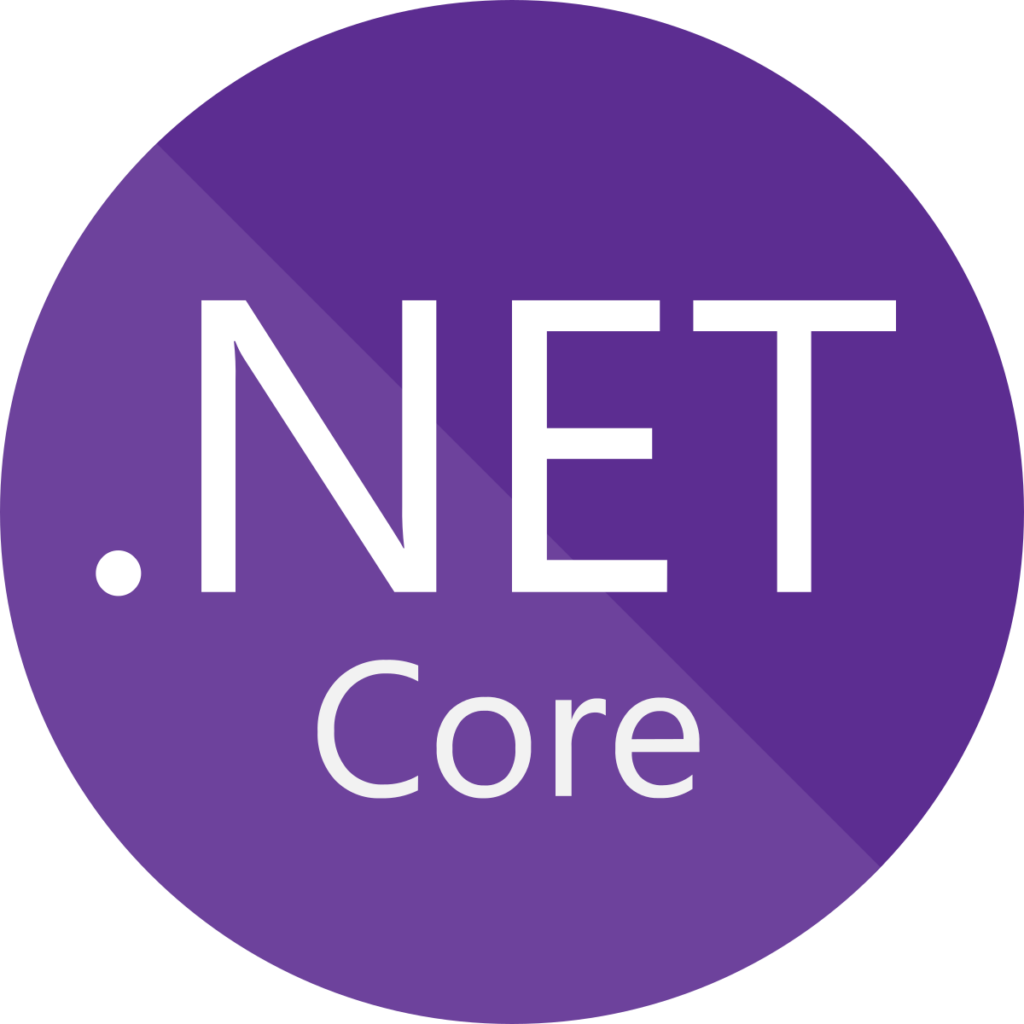
18. Frequently Asked Questions (FAQs) with Answers
Q: Can You Provide an Example of Using Dependency Injection in Dot Net Core 7?
Example: Absolutely! Consider the following example of using DI in a controller:
csharp Copy code
public class SampleController : ControllerBase
{
private readonly ILogger<SampleController> _logger;
public SampleController(ILogger<SampleController> logger)
{
_logger = logger;
}
[HttpGet]
public IActionResult Get()
{
_logger.LogInformation("SampleController Get method called.");
return Ok("Hello, Dot Net Core 7!");
}
}
In this example, the SampleController
class receives an instance of ILogger<SampleController>
through DI, allowing it to log information.
Q: Is Entity Framework Core Suitable for Large-Scale Applications?
Example: Absolutely! Entity Framework Core is designed to handle large-scale applications efficiently. For example, optimize database queries using EF Core’s LINQ to Entities:
csharp Copy code
var products = context.Products
.Where(p => p.Price > 50)
.OrderByDescending(p => p.Price)
.Take(10)
.ToList();
In this example, the LINQ query retrieves the top 10 products with prices greater than 50 from the database.
Q: How Can I Deploy a Dot Net Core 7 Application to Azure?
Example: Deploying a Dot Net Core 7 application to Azure is straightforward. For example, you can use Azure App Service for easy deployment:
- Create an Azure App Service instance.
- Use Azure DevOps or GitHub Actions for continuous integration and deployment.
- Deploy the application using the Azure CLI or Azure Portal.
Q: Is Dot Net Core 7 Suitable for Game Development?
Example: While Dot Net Core 7 is primarily focused on web, cloud, and mobile applications, it can be used for game development. For example, Unity, a popular game engine, supports .NET Core scripting, allowing you to build games with C# and .NET Core.
Q: Can You Explain How to Use Configuration in Dot Net Core 7?
Example: Certainly! Dot Net Core 7 provides a flexible configuration system. For example, use appsettings.json to configure settings:
json Copy code
{
"Logging": {
"LogLevel": "Debug"
},
"MyAppSettings": {
"ApiKey": "my-api-key",
"ConnectionStrings": {
"DefaultConnection": "my-connection-string"
}
}
}
In this example, the MyAppSettings
section contains API key and connection string configurations.
Q: How Can I Improve Performance in Dot Net Core 7 Applications?
Example: Improving performance in Dot Net Core 7 applications involves various optimizations. For example, enable response caching to reduce server load:
csharp Copy code
[ResponseCache(Duration = 3600)]
public IActionResult Get()
{
// Your action logic here
}
In this example, the response from the Get
action is cached for one hour.
Conclusion:
Congratulations! You’ve learned the essential Dot Net Core 7 interview questions with practical examples. Armed with this knowledge, you’ll confidently impress interviewers and demonstrate your expertise in Dot Net Core 7. Keep exploring the framework’s features, practicing coding, and stay updated with the latest advancements to excel in your Dot Net Core 7 journey.
Thank You,
If you are searching how to find best home cleaning services in your area, Please follow instructions
Hi, this is a comment.
To get started with moderating, editing, and deleting comments, please visit the Comments screen in the dashboard.
Commenter avatars come from Gravatar.
Like it.
Question are so helpful.
Good questions
It’s good and helpful 💯